Nanostores in Astro:
A Multi-Framework Adventure
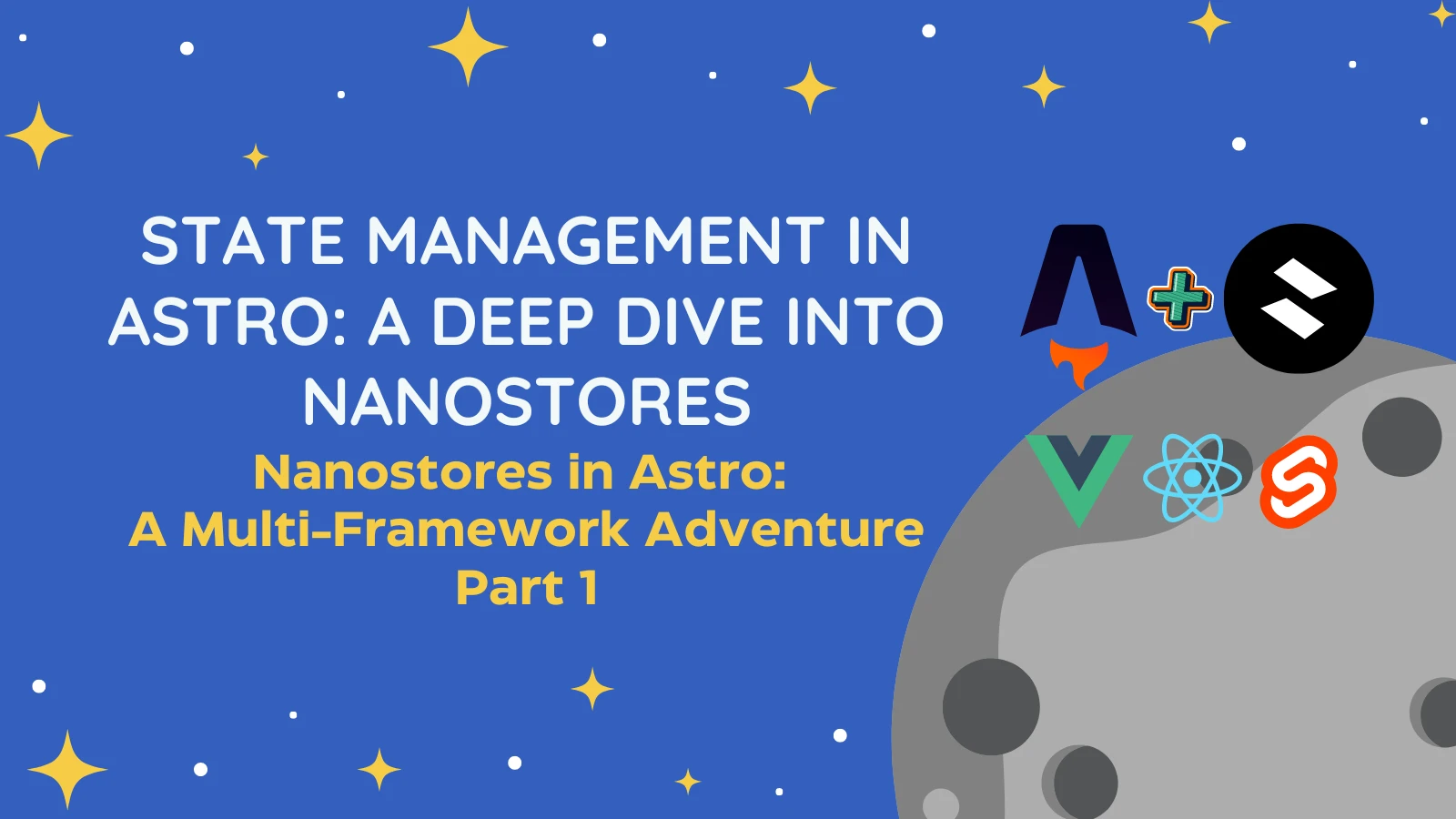
Preface: Handling State in Astro
Welcome to the first article in our series "Nanostores in Astro: A Multi-Framework Adventure". In this series, we'll explore how to effectively manage state in Astro projects, especially when working with multiple frameworks. Our journey begins with an introduction to state management in Astro and how Nanostores can simplify this process.
Astro's component islands architecture allows us to use different frameworks within the same project. However, this flexibility can make state management challenging. That's where Nanostores comes in, offering a lightweight and framework-agnostic solution.
Installing Nanostores
npm install nanostores
npm install @nanostores/react # For React
npm install @nanostores/vue # For Vue
pnpm add nanostores
pnpm add @nanostores/react # For React
pnpm add @nanostores/vue # For Vue
yarn add nanostores
yarn add @nanostores/react # For React
yarn add @nanostores/vue # For Vue
Note: Svelte doesn't require a separate integration package.
Basic Nanostores Usage
import { atom } from 'nanostores'
export const count = atom(0)
export function increment() {
count.set(count.get() + 1)
}
export function decrement() {
count.set(count.get() - 1)
}
---
import { atom, map } from 'nanostores'
const count = atom(0)
const user = map({ name: 'Astro Fan', loggedIn: false })
---
<button id="counter">Count: 0</button>
<div id="user">Welcome, guest!</div>
<script>
import { count, user } from './stores.js'
const counterBtn = document.getElementById('counter')
const userDiv = document.getElementById('user')
count.subscribe(value => {
counterBtn.textContent = `Count: ${value}`
})
</script>
import { useStore } from '@nanostores/react'
import { count } from './stores'
export function Counter() {
const $count = useStore(count)
return <button onClick={() => count.set($count + 1)}>Count: {$count}</button>
}
<script>
import { count } from './stores'
</script>
<button on:click={() => $count++}>
Count: {$count}
</button>
<script setup>
import { useStore } from '@nanostores/vue'
import { count } from './stores'
const $count = useStore(count)
</script>
<button @click="$count++">
Count: {{ $count }}
</button>
What's Next?
In the full article, we'll dive deeper into Nanostores, exploring advanced usage patterns, best practices for state management in Astro, and how to effectively share state between different framework components.
We'll also look at real-world examples and discuss the benefits and potential pitfalls of using Nanostores in multi-framework Astro projects.
Resources
- Full Article: Read the complete guide here
- GitHub Repository: View the code examples
I'm persistent